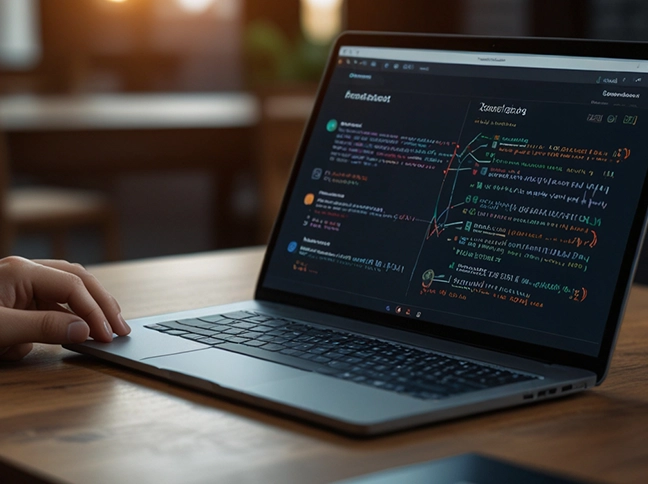
How to Implement a Simple Smart Contract on Ethereum
Smart contracts have become one of the most revolutionary aspects of blockchain technology, particularly on platforms like Ethereum. They allow for decentralized, self-executing agreements coded to operate without the need for a third party. Learning how to implement a simple smart contract on Ethereum is a valuable skill for those diving into the world of blockchain development. This article provides a detailed guide on creating a basic smart contract, walking you through each step to ensure a smooth start.
What is a Smart Contract?
A smart contract is a self-executing contract with the terms of the agreement directly written into code. On the Ethereum blockchain, these contracts are deployed and executed automatically, reducing the need for intermediaries. They can be used in various industries, from finance to supply chain management, automating transactions and verifying conditions.
Getting Started with Ethereum Smart Contracts
Before implementing a smart contract on Ethereum, it's crucial to set up a development environment. Here’s what you’ll need:
- Node.js and npm: JavaScript runtime and package manager.
- Truffle Suite: A popular development framework for Ethereum.
- MetaMask: A browser-based wallet to interact with the Ethereum blockchain.
- Remix IDE: An online tool to write, deploy, and test smart contracts using Solidity, the primary programming language for Ethereum smart contracts.
Step-by-Step Guide to Implementing a Simple Smart Contract
Step 1: Setting Up MetaMask and Test Network
- Install MetaMask: Download MetaMask as a browser extension from metamask.io.
- Set Up a Test Network: MetaMask allows you to connect to the Ethereum mainnet or various testnets. For this guide, use the Ropsten or Rinkeby testnet to avoid real transaction costs. Choose a network by clicking the network dropdown at the top of MetaMask.
- Get Test Ether: Use a faucet to get free test Ether for the Ropsten or Rinkeby testnet. You can find these faucets online by searching "Ropsten faucet" or "Rinkeby faucet."
Step 2: Write the Smart Contract with Solidity
Open Remix IDE: Go to remix.ethereum.org to start coding your smart contract. Remix is an online Solidity compiler and development environment.
Create a New Solidity File: In Remix, create a new file named SimpleContract.sol
.
Write the Smart Contract Code:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 storedData;
function set(uint256 x) public {
storedData = x;
}
function get() public view returns (uint256) {
return storedData;
}
}
- Explanation: This Solidity contract stores a single unsigned integer (
uint256
). Theset
function allows users to store a value, and theget
function retrieves it.
Compile the Contract: In Remix, select the "Solidity Compiler" tab and click the "Compile" button. Make sure the version of Solidity specified in your contract (e.g., ^0.8.0
) matches the compiler version.
Step 3: Deploy the Contract on the Ethereum Testnet
- Select Injected Web3 as Environment: In the "Deploy & Run Transactions" tab of Remix, choose "Injected Web3." This will connect Remix to MetaMask.
- Deploy the Contract: Click the "Deploy" button in Remix. MetaMask will prompt you to confirm the transaction. Since you're using a test network, this process will not cost real Ether.
- Interact with the Contract: After deployment, you can interact with the contract through the functions listed in the "Deployed Contracts" section in Remix. Use the
set
function to store a number and theget
function to retrieve it.
Step 4: Testing and Interacting with the Contract
- Testing: In Remix, you can input values in the
set
function and callget
to ensure your smart contract is working as intended. - Debugging: Remix provides debugging tools to track the execution of your contract and diagnose any issues.
Additional Considerations
- Security: Always test smart contracts on a test network before deploying them on the Ethereum mainnet to avoid potential errors and security vulnerabilities.
- Gas Fees: Every transaction on the Ethereum network requires a fee, known as "gas." The complexity of your contract affects gas costs, so keep smart contracts simple and efficient.
Frequently Asked Questions (FAQ)
1. What is Solidity?
Solidity is the programming language used to write smart contracts on the Ethereum blockchain. It is a statically-typed language inspired by JavaScript, Python, and C++.
2. Can I test smart contracts without using real Ether?
Yes, you can use test networks like Ropsten or Rinkeby to test your smart contracts using free test Ether obtained from faucets.
3. Is it possible to modify a smart contract after deployment?
No, once a smart contract is deployed on the blockchain, it is immutable. If changes are necessary, you need to deploy a new contract.
4. What are gas fees in Ethereum?
Gas fees are the costs required to execute transactions or smart contracts on the Ethereum network. They compensate miners for the computational power required to process transactions.
5. How secure are smart contracts?
Smart contracts are secure when properly coded. However, coding errors or vulnerabilities can lead to exploits. It’s crucial to thoroughly test and audit smart contracts before deployment.
Conclusion
Implementing a simple smart contract on Ethereum is an excellent way to get started with blockchain development. With tools like MetaMask and Remix IDE, you can write, test, and deploy smart contracts efficiently. While this guide covers the basics, there is much more to learn about optimizing smart contracts, enhancing security, and integrating with decentralized applications (dApps). As the blockchain ecosystem grows, mastering smart contract development will open new opportunities.